Given the root
of a binary tree, check whether it is a mirror of itself (i.e., symmetric around its center).
Example 1:
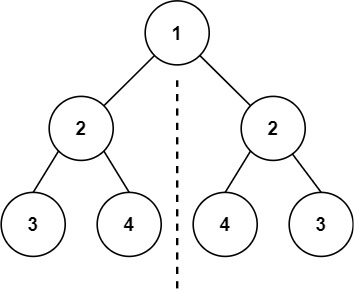
Input: root = [1,2,2,3,4,4,3] Output: true
Example 2:
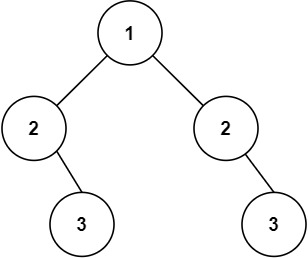
Input: root = [1,2,2,null,3,null,3] Output: false
Solution:
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode() {}
* TreeNode(int val) { this.val = val; }
* TreeNode(int val, TreeNode left, TreeNode right) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
class Solution {
public boolean isSymmetric(TreeNode root) {
return isMirror(root, root);
}
public boolean isMirror(TreeNode node1, TreeNode node2){
if(node1 == null && node2 == null) return true;
if(node1 == null || node2 == null) return false;
return (node1.val==node2.val) && isMirror(node1.left,node2.right) && isMirror(node1.right,node2.left);
}
}
Explanation:
We are taking that same root twice and traversing one to left half of the tree and other root to second half of the tree and comparing if the values are equal or not.
No comments:
Post a Comment